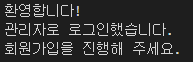
std::pair
https://en.cppreference.com/w/cpp/utility/pair
std::pair - cppreference.com
template< class T1, class T2 > struct pair; std::pair is a class template that provides a way to store two heterogeneous objects as a single unit. A pair is a specific case of a std::tuple with two elements. If neither T1 nor T2 is a possibly c
en.cppreference.com
#include <iostream>
#include <utility>
#include <string>
std::pair<bool, std::string> Login(int userId)
{
if (userId == -1) return {false, "회원가입을 진행해 주세요."};
else if (userId >= 10000) return {true, "관리자로 로그인했습니다."};
return {true, "환영합니다!"};
}
int main()
{
// std::pair<T1, T2> : 두 값을 묶을 수 있다.
std::pair<int, std::string> user;
user.first = 0;
user.second = "Kim Nabin";
std::pair<int, std::string> guest = std::make_pair<int, std::string>(-1, "guest");
// C++11부터는 std::make_pair 대신, 초기화 리스트를 사용할 수 있다.
std::pair<int, std::string> admin = {10000, "admin"};
std::cout << Login(1).second << std::endl;
std::cout << Login(10000).second << std::endl;
std::cout << Login(-1).second << std::endl;
return 0;
}
std::tuple
https://en.cppreference.com/w/cpp/utility/tuple
std::tuple - cppreference.com
template< class... Types > class tuple; (since C++11) Class template std::tuple is a fixed-size collection of heterogeneous values. It is a generalization of std::pair. If std::is_trivially_destructible ::value is true for every Ti in Types, the destructor
en.cppreference.com
#include <iostream>
#include <tuple>
#include <string>
int main()
{
// std::tuple<T1, T2, ...> : 값을 묶을 수 있다. 몇 개를 묶든 제한이 없다. (C++ 11)
std::tuple<int, std::string, std::string> user = {1, "cloud9", "Kim Nabin"};
std::tuple<int, int, int, int> test = std::make_tuple(1, 2, 3, 4);
// std::tuple_size<decltype(인스턴스)>::value : tuple 사이즈를 알 수 있다.
auto size = std::tuple_size<decltype(user)>::value;
std::cout << "tuple size : " << size << std::endl;
// 값을 가져올 때는 std::get<인덱스>(인스턴스)를 사용한다.
std::cout << "tuple values : " << std::get<0>(user) << " " << std::get<1>(user)
<< " " << std::get<2>(user) << " " << std::endl;
// structured binding (C++ 17)
auto [num, id, name] = user;
std::cout << "tuple values with structured binding"
<< num << " " << id << " " << name << std::endl;
return 0;
}
+) optional, variant 추가 예정. vscode가 C++17 빌드하지 못하고 있다. 😅