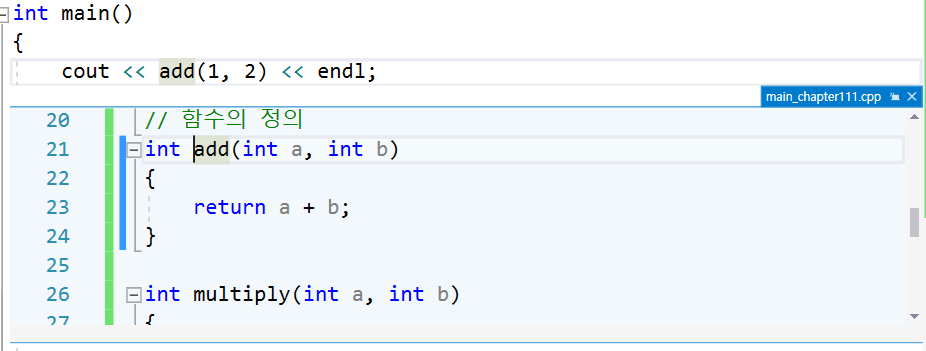
연산자와의 첫 만남
리터럴(Literal)
피연산자(Operand)
단항(unary), 이항(binary), 삼항(ternary)
#include <iostream>
using namespace std;
int main()
{
int x = 2; // x is a variable, 2 is a literal. / assignment: =
cout << x + 2 << endl; // 피연산자(operand): x, 2
cout << -x << endl; // 단항 연산자
cout << 1 + 2 << endl; // 이항 연산자
int y = (x > 0) ? 1 : 2;// c++의 유일한 삼항 연산자, 조건 연산자
// 괄호가 참이면 왼쪽, 거짓이면 오른쪽 값을 갖게 됨
cout << y << endl;
return 0;
}
기본적인 서식 맞추기
컴파일러가 아닌, 프로그래머의 효율을 높이기 위해
선언과 정의의 분리
선언(Declaration)
정의(Definition)
우클릭 - Peek Definition
해당 기능은 함수가 다른 파일에 있을 때 사용하기 유용하다.
#include <iostream>
using namespace std;
// forward declaration
// 최소한의 정보를 포함하고 있는 prototype을 통해 함수를 선언
int add(int a, int b);
int multiply(int a, int b);
int subtract(int a, int b);
int main()
{
cout << add(1, 2) << endl;
cout << subtract(1, 2) << endl;
return 0;
}
// definition
// 함수의 정의
int add(int a, int b)
{
return a + b;
}
int multiply(int a, int b)
{
return a * b;
}
int subtract(int a, int b)
{
return a - b;
}
헤더파일 만들기
헤더파일에 함수 선언, 새로 생성한 파일에 함수 정의 후 메인 파일에 include
main에 코딩하지 않고, 처음부터 헤더와 cpp 파일로 쪼개고 include하여 사용한다.
헤더 가드가 필요한 이유
똑같은 헤더 파일이 두 번 include 되면... => error!
//#pragma once
#ifndef MY_ADD // 전처리기, if not defined MY_ADD
#define MY_ADD // define MY_ADD
int add(int a, int b)
{
return a + b;
}
#endif // !MY_ADD
#pragma once
#include "add.h"
void doSomething()
{
add(1, 2);
}
두 방법 동일하게 작동한다.
네임스페이스 (명칭 공간)
#include <iostream>
namespace MySpace1
{
namespace InnerSpace
{
int my_function()
{
return 0;
}
}
int doSomething(int a, int b)
{
return a + b;
}
}
int doSomething(int a, int b)
{
return a * b;
}
using namespace std;
int main()
{
using namespace MySpace1::InnerSpace;
cout << doSomething(3, 4) << endl; // 3 * 4
cout << MySpace1::doSomething(3, 4) << endl; // 3 + 4
MySpace1::InnerSpace::my_function();
return 0;
}
전처리기와의 첫 만남
Preprocessor: 문서 편집 기능
자주 사용하지 않지만, 이러한 기능이 있다.
define은 해당 파일 안에서만 효력이 있다.
#include <iostream>
#include <algorithm>
using namespace std;
// MY_NUMBER를 만나면 9로 교체하라.
#define MY_NUMBER 9
#define MAX(a, b) (((a)>(b)) ? (a) : (b))
#define LIKE_APPLE
void doSomething();
int main()
{
cout << MY_NUMBER << endl;
cout << MAX(1 + 3, 2) << endl;
cout << std::max(1 + 3, 2) << endl;
// 빌드 들어가기 전에 결정해 버린다.
// 주로 멀티플랫폼 프로그램, 그래픽 카드 사양 등 체크할 때 사용한다.
// 전처리기 라인에서는 교체하지 않는다.
/*
#ifdef LIKE_APPLE
cout << "Apple " << endl;
#else
cout << "Orange " << endl;
#endif // !LIKE_APPLE
*/
// define은 해당 파일 안에서만 적용된다!
doSomething();
return 0;
}
해당 포스트는 '홍정모의 따라하며 배우는 C++' 강의를 수강하며 개인 백업용으로 메모하였습니다.
인프런: https://www.inflearn.com/course/following-c-plus
홍정모의 따라하며 배우는 C++ - 인프런
만약 C++를 쉽게 배울 수 있다면 배우지 않을 이유가 있을까요? 성공한 프로그래머로써의 경력을 꿈꾸지만 지금은 당장 하루하루 마음이 초조할 뿐인 입문자 분들을 돕기 위해 친절하고 자세하게 설명해드리는 강의입니다. 초보로 시작하더라도 중급을 넘어 고급 프로그래머로 가는 길목에 들어서고 싶으시다면 최고의 디딤돌이 되어드리겠습니다. 여러분의 꿈을 응원합니다! 초급 프로그래밍 언어 C++ 온라인 강의 C++
www.inflearn.com